Can DataIku Output to XML
Options
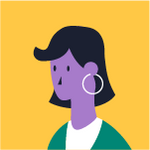
mmc12
Registered Posts: 1 ✭✭✭✭
I need to output a dataset into an XML format for uploading to a service based on an XML template. Is there any way to do this in DataIku?
Thanks.
Thanks.
Tagged:
Answers
-
Hi mmc12,As far as I know, this is not natively supported. However, you can use a python recipe and save your XML file to a folder. The code below illustrates how you can get a handle on a dataset, create a dataframe from it, and then output to XML. I hope it helps!
# -*- coding: utf-8 -*-
import dataiku
import pandas as pd, numpy as np
from dataiku import pandasutils as pdu
from lxml import etree as et
# define your inputs, convert non-string columns into strings
test_dataset = dataiku.Dataset("test_dataset")
df = test_dataset.get_dataframe()
df['column_2'] = df['column_2'].astype(str)
root = et.Element('data')
# iterate over rows and add to the tree
for ix, row in df.iterrows():
item = et.SubElement(root, 'item', attrib=row.to_dict());
# get a handle on the folder to write
xml_files = dataiku.Folder("FOLDER_ID")
with xml_files.get_writer("some_output.xml") as w:
w.write(et.tostring(root, encoding='UTF-8', xml_declaration=False)) -
dhyadav79 Dataiku DSS Core Designer, Dataiku DSS & SQL, Dataiku DSS ML Practitioner, Dataiku DSS Core Concepts, Registered Posts: 21 ✭✭✭✭Options
Nice Code,
Very helpful. Thank you very much.